BCI Kickstarter #05 : Signal Processing in Python: Shaping EEG Data for BCI Applications
Welcome back to our BCI crash course! We've covered the fundamentals of BCIs, explored the brain's electrical activity, and equipped ourselves with the essential Python libraries for BCI development. Now, it's time to roll up our sleeves and dive into the practical world of signal processing. In this blog, we will transform raw EEG data into a format primed for BCI applications using MNE-Python. We will implement basic filters, create epochs around events, explore time-frequency representations, and learn techniques for removing artifacts. To make this a hands-on experience, we will work with the MNE sample dataset, a combined EEG and MEG recording from an auditory and visual experiment.

Getting Ready to Process: Load the Sample Dataset
First, let's load the sample dataset. If you haven't already, make sure you have MNE-Python installed (using conda install -c conda-forge mne). Then, run the following code:
import mne
# Load the sample dataset
data_path = mne.datasets.sample.data_path()
raw_fname = data_path + '/MEG/sample/sample_audvis_filt-0-40_raw.fif'
raw = mne.io.read_raw_fif(raw_fname, preload=True)
# Set the EEG reference to the average
raw.set_eeg_reference('average')
This code snippet loads the EEG data from the sample dataset into a raw object, ready for our signal processing adventures.
Implementing Basic Filters: Refining the EEG Signal
Raw EEG data is often contaminated by noise and artifacts from various sources, obscuring the true brain signals we're interested in. Filtering is a fundamental signal processing technique that allows us to selectively remove unwanted frequencies from our EEG signal.
Applying Filters with MNE: Sculpting the Frequency Landscape
MNE-Python provides a simple yet powerful interface for applying different types of filters to our EEG data using the raw.filter() function. Let's explore the most common filter types:
- High-Pass Filtering: Removes slow drifts and DC offsets, often caused by electrode movement or skin potentials. These low-frequency components can distort our analysis and make it difficult to identify event-related brain activity. Apply a high-pass filter with a cutoff frequency of 0.1 Hz to our sample data using:
raw_highpass = raw.copy().filter(l_freq=0.1, h_freq=None)
- Low-Pass Filtering: Removes high-frequency noise, which can originate from muscle activity or electrical interference. This noise can obscure the slower brain rhythms we're often interested in, such as alpha or beta waves. Apply a low-pass filter with a cutoff frequency of 30 Hz using:
raw_lowpass = raw.copy().filter(l_freq=None, h_freq=30)
- Band-Pass Filtering: Combines high-pass and low-pass filtering to isolate a specific frequency band. This is useful when we're interested in analyzing activity within a particular frequency range, such as the alpha band (8-12 Hz), which is associated with relaxed wakefulness. Apply a band-pass filter to isolate the alpha band using:
raw_bandpass = raw.copy().filter(l_freq=8, h_freq=12)
- Notch Filtering: Removes a narrow band of frequencies, typically used to eliminate power line noise (50/60 Hz) or other specific interference. This noise can create rhythmic artifacts in our data that can interfere with our analysis. Apply a notch filter at 50 Hz using:
raw_notch = raw.copy().notch_filter(freqs=50)
Visualizing Filtered Data: Observing the Effects
To see how filtering shapes our EEG signal, let's visualize the results using MNE-Python's plotting functions:
- Time-Domain Plots: Plot the raw and filtered EEG traces in the time domain using raw.plot(), raw_highpass.plot(), etc. Observe how the different filters affect the appearance of the signal.
- PSD Plots: Visualize the power spectral density (PSD) of the raw and filtered data using raw.plot_psd(), raw_highpass.plot_psd(), etc. Notice how filtering modifies the frequency content of the signal, attenuating power in the filtered bands.
Experiment and Explore: Shaping Your EEG Soundscape
Now it's your turn! Experiment with applying different filter settings to the sample dataset. Change the cutoff frequencies, try different filter types, and observe how the resulting EEG signal is transformed. This hands-on exploration will give you a better understanding of how filtering can be used to refine EEG data for BCI applications.
Epoching and Averaging: Extracting Event-Related Brain Activity
Filtering helps us refine the overall EEG signal, but for many BCI applications, we're interested in how the brain responds to specific events, such as the presentation of a stimulus or a user action. Epoching and averaging are powerful techniques that allow us to isolate and analyze event-related brain activity.
What are Epochs? Time-Locked Windows into Brain Activity
An epoch is a time-locked segment of EEG data centered around a specific event. By extracting epochs, we can focus our analysis on the brain's response to that event, effectively separating it from ongoing background activity.
Finding Events: Marking Moments of Interest
The sample dataset includes dedicated event markers, indicating the precise timing of each stimulus presentation and button press. We can extract these events using the mne.find_events() function:
events = mne.find_events(raw, stim_channel='STI 014')
This code snippet identifies the event markers from the STI 014 channel, commonly used for storing event information in EEG recordings.
Creating Epochs with MNE: Isolating Event-Related Activity
Now, let's create epochs around the events using the mne.Epochs() function:
# Define event IDs for the auditory stimuli
event_id = {'left/auditory': 1, 'right/auditory': 2}
# Set the epoch time window
tmin = -0.2 # 200 ms before the stimulus
tmax = 0.5 # 500 ms after the stimulus
# Create epochs
epochs = mne.Epochs(raw, events, event_id, tmin, tmax, baseline=(-0.2, 0))
This code creates epochs for the left and right auditory stimuli, spanning a time window from 200 ms before to 500 ms after each stimulus onset. The baseline argument applies baseline correction, subtracting the average activity during the pre-stimulus period (-200 ms to 0 ms) to remove any pre-existing bias.
Visualizing Epochs: Exploring Individual Responses
The epochs.plot() function allows us to explore individual epochs and visually inspect the data for artifacts:
epochs.plot()
This interactive visualization displays each epoch as a separate trace, allowing us to see how the EEG signal changes in response to the stimulus. We can scroll through epochs, zoom in on specific time windows, and identify any trials that contain excessive noise or artifacts.
Averaging Epochs: Revealing Event-Related Potentials
To reveal the consistent brain response to a specific event type, we can average the epochs for that event. This averaging process reduces random noise and highlights the event-related potential (ERP), a characteristic waveform reflecting the brain's processing of the event.
# Average the epochs for the left auditory stimulus
evoked_left = epochs['left/auditory'].average()
# Average the epochs for the right auditory stimulus
evoked_right = epochs['right/auditory'].average()
Plotting Evoked Responses: Visualizing the Average Brain Response
MNE-Python provides a convenient function for plotting the average evoked response:
evoked_left.plot()
evoked_right.plot()
This visualization displays the average ERP waveform for each auditory stimulus condition, showing how the brain's electrical activity changes over time in response to the sounds.
Analyze and Interpret: Unveiling the Brain's Auditory Processing
Now it's your turn! Analyze the evoked responses for the left and right auditory stimuli. Compare the waveforms, looking for differences in amplitude, latency, or morphology. Can you identify any characteristic ERP components, such as the N100 or P300? What do these differences tell you about how the brain processes sounds from different spatial locations?
Time-Frequency Analysis: Unveiling Dynamic Brain Rhythms
Epoching and averaging allow us to analyze the brain's response to events in the time domain. However, EEG signals are often non-stationary, meaning their frequency content changes over time. To capture these dynamic shifts in brain activity, we turn to time-frequency analysis.
Time-frequency analysis provides a powerful lens for understanding how brain rhythms evolve in response to events or cognitive tasks. It allows us to see not just when brain activity changes but also how the frequency content of the signal shifts over time.
Wavelet Transform with MNE: A Window into Time and Frequency
The wavelet transform is a versatile technique for time-frequency analysis. It decomposes the EEG signal into a set of wavelets, functions that vary in both frequency and time duration, providing a detailed representation of how different frequencies contribute to the signal over time.
MNE-Python offers the mne.time_frequency.tfr_morlet() function for computing the wavelet transform:
from mne.time_frequency import tfr_morlet
# Define the frequencies of interest
freqs = np.arange(7, 30, 1) # From 7 Hz to 30 Hz in 1 Hz steps
# Set the number of cycles for the wavelets
n_cycles = freqs / 2. # Increase the number of cycles with frequency
# Compute the wavelet transform for the left auditory epochs
power_left, itc_left = tfr_morlet(epochs['left/auditory'], freqs=freqs, n_cycles=n_cycles, use_fft=True, return_itc=True)
# Compute the wavelet transform for the right auditory epochs
power_right, itc_right = tfr_morlet(epochs['right/auditory'], freqs=freqs, n_cycles=n_cycles, use_fft=True, return_itc=True)
This code computes the wavelet transform for the left and right auditory epochs, focusing on frequencies from 7 Hz to 30 Hz. The n_cycles parameter determines the time resolution and frequency smoothing of the transform.
Visualizing Time-Frequency Representations: Spectrograms of Brain Activity
To visualize the time-frequency representations, we can use the mne.time_frequency.AverageTFR.plot() function:
power_left.plot([0], baseline=(-0.2, 0), mode='logratio', title="Left Auditory Stimulus")
power_right.plot([0], baseline=(-0.2, 0), mode='logratio', title="Right Auditory Stimulus")
This code displays spectrograms, plots that show the power distribution across frequencies over time. The baseline argument normalizes the power values to the pre-stimulus period, highlighting event-related changes.
Interpreting Time-Frequency Results
Time-frequency representations reveal how the brain's rhythmic activity evolves over time. Increased power in specific frequency bands after the stimulus can indicate the engagement of different cognitive processes. For example, we might observe increased alpha power during sensory processing or enhanced beta power during attentional engagement.
Discovering Dynamic Brain Patterns
Now, explore the time-frequency representations for the left and right auditory stimuli. Look for changes in power across different frequency bands following the stimulus onset. Do you observe any differences between the two conditions? What insights can you gain about the dynamic nature of auditory processing in the brain?
Artifact Removal Techniques: Cleaning Up Noisy Data
Even after careful preprocessing, EEG data can still contain artifacts that distort our analysis and hinder BCI performance. This section explores techniques for identifying and removing these unwanted signals, ensuring cleaner and more reliable data for our BCI applications.
Identifying Artifacts: Spotting the Unwanted Guests
- Visual Inspection: We can visually inspect raw EEG traces (raw.plot()) and epochs (epochs.plot()) to identify obvious artifacts, such as eye blinks, muscle activity, or electrode movement.
- Automated Methods: Algorithms can automatically detect specific artifact patterns based on their characteristic features, such as the high amplitude and slow frequency of eye blinks.
Rejecting Noisy Epochs: Discarding the Troublemakers
One approach to artifact removal is to simply discard noisy epochs. We can set rejection thresholds based on signal amplitude using the reject parameter in the mne.Epochs() function:
# Set rejection thresholds for EEG and EOG channels
reject = dict(eeg=150e-6) # Reject epochs with EEG activity exceeding 150 µV
# Create epochs with rejection criteria
epochs = mne.Epochs(raw, events, event_id, tmin, tmax, baseline=(-0.2, 0), reject=reject)
This code rejects epochs where the peak-to-peak amplitude of the EEG signal exceeds 150 µV, helping to eliminate trials contaminated by high-amplitude artifacts.
Independent Component Analysis (ICA): Unmixing the Signal Cocktail
Independent component analysis (ICA) is a powerful technique for separating independent sources of activity within EEG data. It assumes that the recorded EEG signal is a mixture of independent signals originating from different brain regions and artifact sources.
MNE-Python provides the mne.preprocessing.ICA() function for performing ICA:
from mne.preprocessing import ICA
# Create an ICA object
ica = ICA(n_components=20, random_state=97)
# Fit the ICA to the EEG data
ica.fit(raw)
We can then visualize the independent components using ica.plot_components() and identify components that correspond to artifacts based on their characteristic time courses and scalp topographies. Once identified, these artifact components can be removed from the data, leaving behind cleaner EEG signals.
Experiment and Explore: Finding the Right Cleaning Strategy
Artifact removal is an art as much as a science. Experiment with different artifact removal techniques and settings to find the best strategy for your specific dataset and BCI application. Visual inspection, rejection thresholds, and ICA can be combined to achieve optimal results.
Mastering the Art of Signal Processing
We've journeyed through the essential steps of signal processing in Python, transforming raw EEG data into a form ready for BCI applications. We've implemented basic filters, extracted epochs, explored time-frequency representations, and tackled artifact removal, building a powerful toolkit for shaping and refining brainwave data.
Remember, careful signal processing is the foundation for reliable and accurate BCI development. By mastering these techniques, you're well on your way to creating innovative applications that translate brain activity into action.
Resources and Further Reading
- MIT course on Signals and Systems: https://ocw.mit.edu/courses/res-6-007-signals-and-systems-spring-2011/
- Book: Smith, S. W. (2002). The scientist and engineer's guide to digital signal processing. California Technical Publishing.
From Processed Signals to Intelligent Algorithms: The Next Level
This concludes our deep dive into signal processing techniques using Python and MNE-Python. You've gained valuable hands-on experience in cleaning up, analyzing, and extracting meaningful information from EEG data, setting the stage for the next exciting phase of our BCI journey.
In the next post, we'll explore the world of machine learning for BCI, where we'll train algorithms to decode user intent, predict mental states, and control external devices directly from brain signals. Get ready to witness the magic of intelligent algorithms transforming processed brainwaves into real-world BCI applications!

Welcome back to our BCI crash course! Over the past eight blogs, we have explored the fascinating intersection of neuroscience, engineering, and machine learning, from the fundamental concepts of BCIs to the practical implementation of real-world applications. In this final installment, we will shift our focus to the future of BCI, delving into advanced topics and research directions that are pushing the boundaries of mind-controlled technology. Get ready to explore the exciting possibilities of hybrid BCIs, adaptive algorithms, ethical considerations, and the transformative potential that lies ahead for this groundbreaking field.
Hybrid BCIs: Combining Paradigms for Enhanced Performance
As we've explored in previous posts, different BCI paradigms leverage distinct brain signals and have their strengths and limitations. Motor imagery BCIs excel at decoding movement intentions, P300 spellers enable communication through attention-based selections, and SSVEP BCIs offer high-speed control using visual stimuli.
What are Hybrid BCIs? Synergy of Brain Signals
Hybrid BCIs combine multiple BCI paradigms, integrating different brain signals to create more robust, versatile, and user-friendly systems. Imagine a BCI that leverages both motor imagery and SSVEP to control a robotic arm with greater precision and flexibility, or a system that combines P300 with error-related potentials (ErrPs) to improve the accuracy and speed of a speller.
Benefits of Hybrid BCIs: Unlocking New Possibilities
Hybrid BCIs offer several advantages over single-paradigm systems:
- Improved Accuracy and Reliability: Combining complementary brain signals can enhance the signal-to-noise ratio and reduce the impact of individual variations in brain activity, leading to more accurate and reliable BCI control.
- Increased Flexibility and Adaptability: Hybrid BCIs can adapt to different user needs, tasks, and environments by dynamically switching between paradigms or combining them in a way that optimizes performance.
- Richer and More Natural Interactions: Integrating multiple BCI paradigms opens up possibilities for creating more intuitive and natural BCI interactions, allowing users to control devices with a greater range of mental commands.
Examples of Hybrid BCIs: Innovations in Action
Research is exploring various hybrid BCI approaches:
- Motor Imagery + SSVEP: Combining motor imagery with SSVEP can enhance the control of robotic arms. Motor imagery provides continuous control signals for movement direction, while SSVEP enables discrete selections for grasping or releasing objects.
- P300 + ErrP: Integrating P300 with ErrPs, brain signals that occur when we make errors, can improve speller accuracy. The P300 is used to select letters, while ErrPs can be used to automatically correct errors, reducing the need for manual backspacing.
Adaptive BCIs: Learning and Evolving with the User
One of the biggest challenges in BCI development is the inherent variability in brain signals. A BCI system that works perfectly for one user might perform poorly for another, and even a single user's brain activity can change over time due to factors like learning, fatigue, or changes in attention. This is where adaptive BCIs come into play, offering a dynamic and personalized approach to brain-computer interaction.
The Need for Adaptation: Embracing the Brain's Dynamic Nature
BCI systems need to adapt to several factors:
- Changes in User Brain Activity: Brain signals are not static. They evolve as users learn to control the BCI, become fatigued, or shift their attention. An adaptive BCI can track these changes and adjust its processing accordingly.
- Variations in Signal Quality and Noise: EEG recordings can be affected by various sources of noise, from muscle artifacts to environmental interference. An adaptive BCI can adjust its filtering and artifact rejection parameters to maintain optimal signal quality.
- Different User Preferences and Skill Levels: BCI users have different preferences for control strategies, feedback modalities, and interaction speeds. An adaptive BCI can personalize its settings to match each user's individual needs and skill level.
Methods for Adaptation: Tailoring BCIs to the Individual
Various techniques can be employed to create adaptive BCIs:
- Machine Learning Adaptation: Machine learning algorithms, such as those used for classification, can be trained to continuously learn and update the BCI model based on the user's brain data. This allows the BCI to adapt to changes in brain patterns over time and improve its accuracy and responsiveness.
- User Feedback Adaptation: BCIs can incorporate user feedback, either explicitly (through direct input) or implicitly (by monitoring performance and user behavior), to adjust parameters and optimize the interaction. For example, if a user consistently struggles to control a motor imagery BCI, the system could adjust the classification thresholds or provide more frequent feedback to assist them.
Benefits of Adaptive BCIs: A Personalized and Evolving Experience
Adaptive BCIs offer significant advantages:
- Enhanced Usability and User Experience: By adapting to individual needs and preferences, adaptive BCIs can become more intuitive and easier to use, reducing user frustration and improving the overall experience.
- Improved Long-Term Performance and Reliability: Adaptive BCIs can maintain high levels of performance and reliability over time by adjusting to changes in brain activity and signal quality.
- Personalized BCIs: Adaptive algorithms can tailor the BCI to each user's unique brain patterns, preferences, and abilities, creating a truly personalized experience.
Ethical Considerations: Navigating the Responsible Development of BCI
As BCI technology advances, it's crucial to consider the ethical implications of its development and use. BCIs have the potential to profoundly impact individuals and society, raising questions about privacy, autonomy, fairness, and responsibility.
Introduction: Ethics at the Forefront of BCI Innovation
Ethical considerations should be woven into the fabric of BCI research and development, guiding our decisions and ensuring that this powerful technology is used for good.
Key Ethical Concerns: Navigating a Complex Landscape
- Privacy and Data Security: BCIs collect sensitive brain data, raising concerns about privacy violations and potential misuse. Robust data security measures and clear ethical guidelines are crucial for protecting user privacy and ensuring responsible data handling.
- Agency and Autonomy: BCIs have the potential to influence user thoughts, emotions, and actions. It's essential to ensure that BCI use respects user autonomy and agency, avoiding coercion, manipulation, or unintended consequences.
- Bias and Fairness: BCI algorithms can inherit biases from the data they are trained on, potentially leading to unfair or discriminatory outcomes. Addressing these biases and developing fair and equitable BCI systems is essential for responsible innovation.
- Safety and Responsibility: As BCIs become more sophisticated and integrated into critical applications like healthcare and transportation, ensuring their safety and reliability is paramount. Clear lines of responsibility and accountability need to be established to mitigate potential risks and ensure ethical use.
Guidelines and Principles: A Framework for Responsible BCI
Efforts are underway to establish ethical guidelines and principles for BCI research and development. These guidelines aim to promote responsible innovation, protect user rights, and ensure that BCI technology benefits society as a whole.
Current Challenges and Future Prospects: The Road Ahead for BCI
While BCI technology has made remarkable progress, several challenges remain to be addressed before it can fully realize its transformative potential. However, the future of BCI is bright, with exciting possibilities on the horizon for enhancing human capabilities, restoring lost function, and improving lives.
Technical Challenges: Overcoming Roadblocks to Progress
- Signal Quality and Noise: Non-invasive BCIs, particularly those based on EEG, often suffer from low signal-to-noise ratios. Improving signal quality through advanced electrode designs, noise reduction algorithms, and a better understanding of brain signals is crucial for enhancing BCI accuracy and reliability.
- Robustness and Generalizability: Current BCI systems often work well in controlled laboratory settings but struggle to perform consistently across different users, environments, and tasks. Developing more robust and generalizable BCIs is essential for wider adoption and real-world applications.
- Long-Term Stability: Maintaining the long-term stability and performance of BCI systems, especially for implanted devices, is a significant challenge. Addressing issues like biocompatibility, signal degradation, and device longevity is crucial for ensuring the viability of invasive BCIs.
Future Directions: Expanding the BCI Horizon
- Non-invasive Advancements: Research is focusing on developing more sophisticated and user-friendly non-invasive BCI systems. Advancements in EEG technology, including dry electrodes, high-density arrays, and mobile brain imaging, hold promise for creating more portable, comfortable, and accurate non-invasive BCIs.
- Clinical Applications: BCIs are showing increasing promise for clinical applications, such as restoring lost motor function in individuals with paralysis, assisting in stroke rehabilitation, and treating neurological disorders like epilepsy and Parkinson's disease. Ongoing research and clinical trials are paving the way for wider adoption of BCIs in healthcare.
- Cognitive Enhancement: BCIs have the potential to enhance cognitive abilities, such as memory, attention, and learning. Research is exploring ways to use BCIs for cognitive training and to develop brain-computer interfaces that can augment human cognitive function.
- Brain-to-Brain Communication: One of the most futuristic and intriguing directions in BCI research is the possibility of direct brain-to-brain communication. Studies have already demonstrated the feasibility of transmitting simple signals between brains, opening up possibilities for collaborative problem-solving, enhanced empathy, and new forms of communication.
Resources for Further Learning and Development
- Brain-Computer Interface Wiki
- Research Journals and Conferences:some text
- Journal of Neural Engineering: https://iopscience.iop.org/journal/1741-2560 - A leading journal for BCI research and related fields.
- Brain-Computer Interfaces: https://www.tandfonline.com/toc/tbci20/current - A dedicated journal focusing on advances in BCI technology and applications.
Embracing the Transformative Power of BCI
From hybrid systems to adaptive algorithms, ethical considerations, and the exciting possibilities of the future, we've explored the cutting edge of BCI technology. This field is rapidly evolving, driven by advancements in neuroscience, engineering, and machine learning.
BCIs hold immense potential to revolutionize how we interact with technology, enhance human capabilities, restore lost function, and improve lives. As we continue to push the boundaries of mind-controlled technology, the future promises a world where our thoughts can seamlessly translate into actions, unlocking new possibilities for communication, control, and human potential.
As we wrap up this course with this final blog article, we hope that you gained an overview as well as practical expertise in the field of BCIs. Please feel free to reach out to us with feedback and areas of improvement. Thank you for reading along so far, and best wishes for further endeavors in your BCI journey!
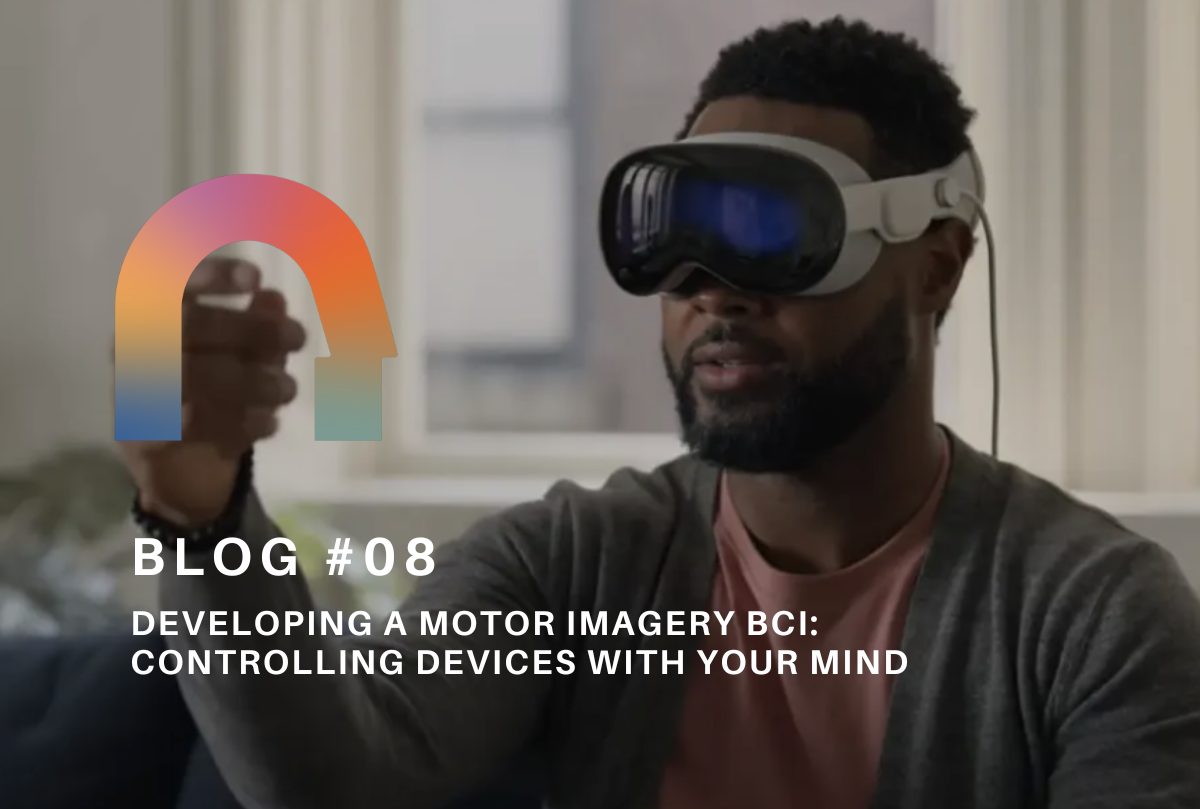
Welcome back to our BCI crash course! We've journeyed from the fundamental concepts of BCIs to the intricacies of brain signals, mastered the art of signal processing, and learned how to train intelligent algorithms to decode those signals. Now, we're ready to tackle a fascinating and powerful BCI paradigm: motor imagery. Motor imagery BCIs allow users to control devices simply by imagining movements. This technology holds immense potential for applications like controlling neuroprosthetics for individuals with paralysis, assisting in stroke rehabilitation, and even creating immersive gaming experiences. In this post, we'll guide you through the step-by-step process of building a basic motor imagery BCI using Python, MNE-Python, and scikit-learn. Get ready to harness the power of your thoughts to interact with technology!
Understanding Motor Imagery: The Brain's Internal Rehearsal
Before we dive into building our BCI, let's first understand the fascinating phenomenon of motor imagery.
What is Motor Imagery? Moving Without Moving
Motor imagery is the mental rehearsal of a movement without actually performing the physical action. It's like playing a video of the movement in your mind's eye, engaging the same neural processes involved in actual execution but without sending the final commands to your muscles.
Neural Basis of Motor Imagery: The Brain's Shared Representations
Remarkably, motor imagery activates similar brain regions and neural networks as actual movement. The motor cortex, the area of the brain responsible for planning and executing movements, is particularly active during motor imagery. This shared neural representation suggests that imagining a movement is a powerful way to engage the brain's motor system, even without physical action.
EEG Correlates of Motor Imagery: Decoding Imagined Movements
Motor imagery produces characteristic changes in EEG signals, particularly over the motor cortex. Two key features are:
- Event-Related Desynchronization (ERD): A decrease in power in specific frequency bands (mu, 8-12 Hz, and beta, 13-30 Hz) over the motor cortex during motor imagery. This decrease reflects the activation of neural populations involved in planning and executing the imagined movement.
- Event-Related Synchronization (ERS): An increase in power in those frequency bands after the termination of motor imagery, as the brain returns to its resting state.
These EEG features provide the foundation for decoding motor imagery and building BCIs that can translate imagined movements into control signals.
Building a Motor Imagery BCI: A Step-by-Step Guide
Now that we understand the neural basis of motor imagery, let's roll up our sleeves and build a BCI that can decode these imagined movements. We'll follow a step-by-step process, using Python, MNE-Python, and scikit-learn to guide us.
1. Loading the Dataset
Choosing the Dataset: BCI Competition IV Dataset 2a
For this project, we'll use the BCI Competition IV dataset 2a, a publicly available EEG dataset specifically designed for motor imagery BCI research. This dataset offers several advantages:
- Standardized Paradigm: The dataset follows a well-defined experimental protocol, making it easy to understand and replicate. Participants were instructed to imagine moving their left or right hand, providing clear labels for our classification task.
- Multiple Subjects: It includes recordings from nine subjects, providing a decent sample size to train and evaluate our BCI model.
- Widely Used: This dataset has been extensively used in BCI research, allowing us to compare our results with established benchmarks and explore various analysis approaches.
You can download the dataset from the BCI Competition IV website (http://www.bbci.de/competition/iv/).
Loading the Data: MNE-Python to the Rescue
Once you have the dataset downloaded, you can load it using MNE-Python's convenient functions. Here's a code snippet to get you started:
import mne
# Set the path to the dataset directory
data_path = '<path_to_dataset_directory>'
# Load the raw EEG data for subject 1
raw = mne.io.read_raw_gdf(data_path + '/A01T.gdf', preload=True)
Replace <path_to_dataset_directory> with the actual path to the directory where you've stored the dataset files. This code loads the data for subject "A01" from the training session ("T").
2. Data Preprocessing: Preparing the Signals for Decoding
Raw EEG data is often noisy and contains artifacts that can interfere with our analysis. Preprocessing is crucial for cleaning up the data and isolating the relevant brain signals associated with motor imagery.
Channel Selection: Focusing on the Motor Cortex
Since motor imagery primarily activates the motor cortex, we'll select EEG channels that capture activity from this region. Key channels include:
- C3: Located over the left motor cortex, sensitive to right-hand motor imagery.
- C4: Located over the right motor cortex, sensitive to left-hand motor imagery.
- Cz: Located over the midline, often used as a reference or to capture general motor activity.
# Select the desired channels
channels = ['C3', 'C4', 'Cz']
# Create a new raw object with only the selected channels
raw_selected = raw.pick_channels(channels)
Filtering: Isolating Mu and Beta Rhythms
We'll apply a band-pass filter to isolate the mu (8-12 Hz) and beta (13-30 Hz) frequency bands, as these rhythms exhibit the most prominent ERD/ERS patterns during motor imagery.
# Apply a band-pass filter from 8 Hz to 30 Hz
raw_filtered = raw_selected.filter(l_freq=8, h_freq=30)
This filtering step removes irrelevant frequencies and enhances the signal-to-noise ratio for detecting motor imagery-related brain activity.
Artifact Removal: Enhancing Data Quality (Optional)
Depending on the dataset and the quality of the recordings, we might need to apply artifact removal techniques. Independent Component Analysis (ICA) is particularly useful for identifying and removing artifacts like eye blinks, muscle activity, and heartbeats, which can contaminate our motor imagery signals. MNE-Python provides functions for performing ICA and visualizing the components, allowing us to select and remove those associated with artifacts. This step can significantly improve the accuracy and reliability of our motor imagery BCI.
3. Epoching and Visualizing: Zooming in on Motor Imagery
Now that we've preprocessed our EEG data, let's create epochs around the motor imagery cues, allowing us to focus on the brain activity specifically related to those imagined movements.
Defining Epochs: Capturing the Mental Rehearsal
The BCI Competition IV dataset 2a includes event markers indicating the onset of the motor imagery cues. We'll use these markers to create epochs, typically spanning a time window from a second before the cue to several seconds after it. This window captures the ERD and ERS patterns associated with motor imagery.
# Define event IDs for left and right hand motor imagery (refer to dataset documentation)
event_id = {'left_hand': 1, 'right_hand': 2}
# Set the epoch time window
tmin = -1 # 1 second before the cue
tmax = 4 # 4 seconds after the cue
# Create epochs
epochs = mne.Epochs(raw_filtered, events, event_id, tmin, tmax, baseline=(-1, 0), preload=True)
Baseline Correction: Removing Pre-Imagery Bias
We'll apply baseline correction to remove any pre-existing bias in the EEG signal, ensuring that our analysis focuses on the changes specifically related to motor imagery.
Visualizing: Inspecting and Gaining Insights
- Plotting Epochs: Use epochs.plot() to visualize individual epochs, inspecting for artifacts and observing the general patterns of brain activity during motor imagery.
- Topographical Maps: Use epochs['left_hand'].average().plot_topomap() and epochs['right_hand'].average().plot_topomap() to visualize the scalp distribution of mu and beta power changes during left and right hand motor imagery. These maps can help validate our channel selection and confirm that the ERD patterns are localized over the expected motor cortex areas.
4. Feature Extraction with Common Spatial Patterns (CSP): Maximizing Class Differences
Common Spatial Patterns (CSP) is a spatial filtering technique specifically designed to extract features that best discriminate between two classes of EEG data. In our case, these classes are left-hand and right-hand motor imagery.
Understanding CSP: Finding Optimal Spatial Filters
CSP seeks to find spatial filters that maximize the variance of one class while minimizing the variance of the other. It achieves this by solving an eigenvalue problem based on the covariance matrices of the two classes. The resulting spatial filters project the EEG data onto a new space where the classes are more easily separable
.
Applying CSP: MNE-Python's CSP Function
MNE-Python's mne.decoding.CSP() function makes it easy to extract CSP features:
from mne.decoding import CSP
# Create a CSP object
csp = CSP(n_components=4, reg=None, log=True, norm_trace=False)
# Fit the CSP to the epochs data
csp.fit(epochs['left_hand'].get_data(), epochs['right_hand'].get_data())
# Transform the epochs data using the CSP filters
X_csp = csp.transform(epochs.get_data())
Interpreting CSP Filters: Mapping Brain Activity
The CSP spatial filters represent patterns of brain activity that differentiate between left and right hand motor imagery. By visualizing these filters, we can gain insights into the underlying neural sources involved in these imagined movements.
Selecting CSP Components: Balancing Performance and Complexity
The n_components parameter in the CSP() function determines the number of CSP components to extract. Choosing the optimal number of components is crucial for balancing classification performance and model complexity. Too few components might not capture enough information, while too many can lead to overfitting. Cross-validation can help us find the optimal balance.
5. Classification with a Linear SVM: Decoding Motor Imagery
Choosing the Classifier: Linear SVM for Simplicity and Efficiency
We'll use a linear Support Vector Machine (SVM) to classify our motor imagery data. Linear SVMs are well-suited for this task due to their simplicity, efficiency, and ability to handle high-dimensional data. They seek to find a hyperplane that best separates the two classes in the feature space.
Training the Model: Learning from Spatial Patterns
from sklearn.svm import SVC
# Create a linear SVM classifier
svm = SVC(kernel='linear')
# Train the SVM model
svm.fit(X_csp_train, y_train)
Hyperparameter Tuning: Optimizing for Peak Performance
SVMs have hyperparameters, like the regularization parameter C, that control the model's complexity and generalization ability. Hyperparameter tuning, using techniques like grid search or cross-validation, helps us find the optimal values for these parameters to maximize classification accuracy.
Evaluating the Motor Imagery BCI: Measuring Mind Control
We've built our motor imagery BCI, but how well does it actually work? Evaluating its performance is crucial for understanding its capabilities and limitations, especially if we envision real-world applications.
Cross-Validation: Assessing Generalizability
To obtain a reliable estimate of our BCI's performance, we'll employ k-fold cross-validation. This technique helps us assess how well our model generalizes to unseen data, providing a more realistic measure of its real-world performance.
from sklearn.model_selection import cross_val_score
# Perform 5-fold cross-validation
scores = cross_val_score(svm, X_csp, y, cv=5)
# Print the average accuracy across the folds
print("Average accuracy: %0.2f" % scores.mean())
Performance Metrics: Beyond Simple Accuracy
- Accuracy: While accuracy, the proportion of correctly classified instances, is a useful starting point, it doesn't tell the whole story. For imbalanced datasets (where one class has significantly more samples than the other), accuracy can be misleading.
- Kappa Coefficient: The Kappa coefficient (κ) measures the agreement between the classifier's predictions and the true labels, taking into account the possibility of chance agreement. A Kappa value of 1 indicates perfect agreement, while 0 indicates agreement equivalent to chance. Kappa is a more robust metric than accuracy, especially for imbalanced datasets.
- Information Transfer Rate (ITR): ITR quantifies the amount of information transmitted by the BCI per unit of time, considering both accuracy and the number of possible choices. A higher ITR indicates a faster and more efficient communication system.
- Sensitivity and Specificity: These metrics provide a more nuanced view of classification performance. Sensitivity measures the proportion of correctly classified positive instances (e.g., correctly identifying left-hand imagery), while specificity measures the proportion of correctly classified negative instances (e.g., correctly identifying right-hand imagery).
Practical Implications: From Benchmarks to Real-World Use
Evaluating a motor imagery BCI goes beyond just looking at numbers. We need to consider the practical implications of its performance:
- Minimum Accuracy Requirements: Real-world applications often have minimum accuracy thresholds. For example, a neuroprosthetic controlled by a motor imagery BCI might require an accuracy of over 90% to ensure safe and reliable operation.
- User Experience: Beyond accuracy, factors like speed, ease of use, and mental effort also contribute to the overall user experience.
Unlocking the Potential of Motor Imagery BCIs
We've successfully built a basic motor imagery BCI, witnessing the power of EEG, signal processing, and machine learning to decode movement intentions directly from brain signals. Motor imagery BCIs hold immense potential for a wide range of applications, offering new possibilities for individuals with disabilities, stroke rehabilitation, and even immersive gaming experiences.
Resources for Further Reading
- Review article: EEG-Based Brain-Computer Interfaces Using Motor-Imagery: Techniques and Challenges https://www.ncbi.nlm.nih.gov/pmc/articles/PMC6471241/
- Review article: A review of critical challenges in MI-BCI: From conventional to deep learning methods https://www.sciencedirect.com/science/article/abs/pii/S016502702200262X
- BCI Competition IV Dataset 2a https://www.bbci.de/competition/iv/desc_2a.pdf
From Motor Imagery to Advanced BCI Paradigms
This concludes our exploration of building a motor imagery BCI. You've gained valuable insights into the neural basis of motor imagery, learned how to extract features using CSP, trained a classifier to decode movement intentions, and evaluated the performance of your BCI model.
In our final blog post, we'll explore the exciting frontier of advanced BCI paradigms and future directions. We'll delve into concepts like hybrid BCIs, adaptive algorithms, ethical considerations, and the ever-expanding possibilities that lie ahead in the world of brain-computer interfaces. Stay tuned for a glimpse into the future of mind-controlled technology!
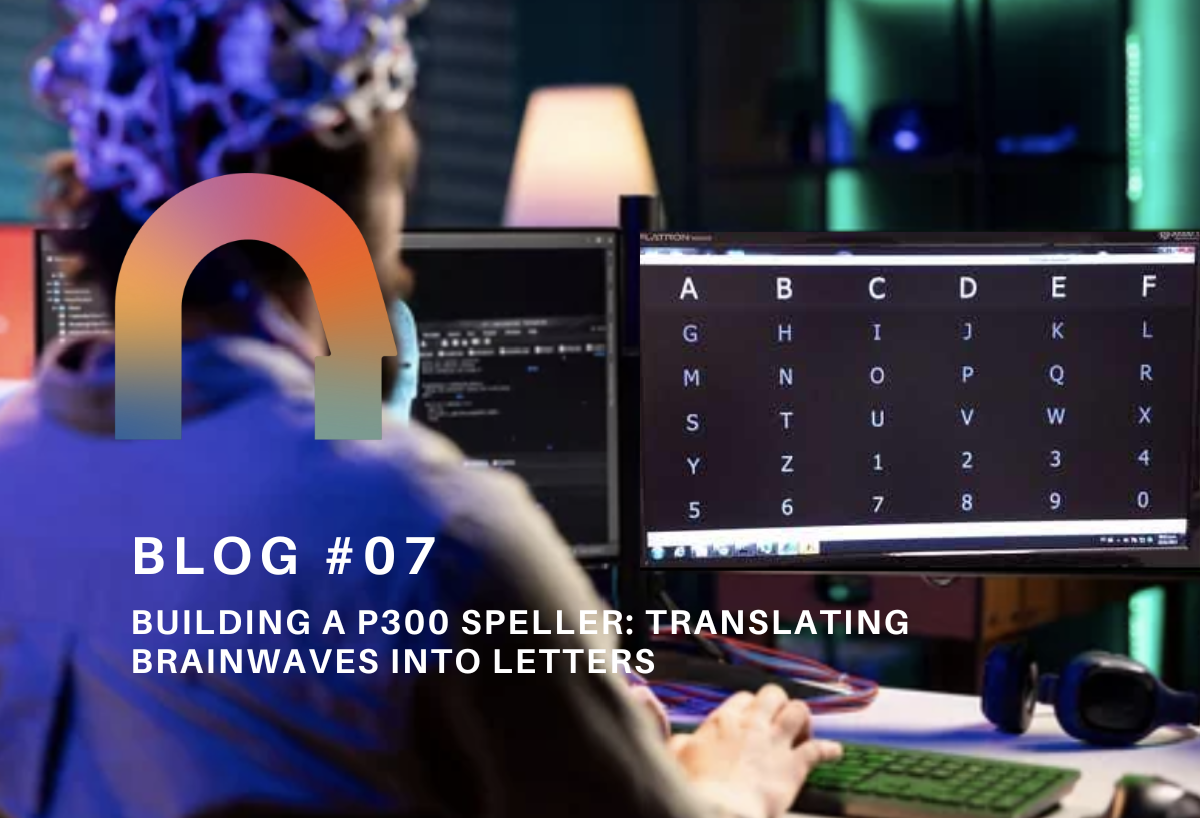
Welcome back to our BCI crash course! We've explored the foundations of BCIs, delved into the intricacies of brain signals, mastered the art of signal processing, and learned how to train intelligent algorithms to decode those signals. Now, we are ready to put all this knowledge into action by building a real-world BCI application: a P300 speller. P300 spellers are a groundbreaking technology that allows individuals with severe motor impairments to communicate by simply focusing their attention on letters on a screen. By harnessing the power of the P300 event-related potential, a brain response elicited by rare or surprising stimuli, these spellers open up a world of communication possibilities for those who might otherwise struggle to express themselves. In this blog, we will guide you through the step-by-step process of building a P300 speller using Python, MNE-Python, and scikit-learn. Get ready for a hands-on adventure in BCI development as we translate brainwaves into letters and words!
Step-by-Step Implementation: A Hands-on BCI Project
1. Loading the Dataset
Introducing the BNCI Horizon 2020 Dataset: A Rich Resource for P300 Speller Development
For this project, we'll use the BNCI Horizon 2020 dataset, a publicly available EEG dataset specifically designed for P300 speller research. This dataset offers several advantages:
- Large Sample Size: It includes recordings from a substantial number of participants, providing a diverse range of P300 responses.
- Standardized Paradigm: The dataset follows a standardized experimental protocol, ensuring consistency and comparability across recordings.
- Detailed Metadata: It provides comprehensive metadata, including information about stimulus presentation, participant responses, and electrode locations.
This dataset is well-suited for our P300 speller project because it provides high-quality EEG data recorded during a classic P300 speller paradigm, allowing us to focus on the core signal processing and machine learning steps involved in building a functional speller.
Loading the Data with MNE-Python: Accessing the Brainwave Symphony
To load the BNCI Horizon 2020 dataset using MNE-Python, you'll need to download the data files from the dataset's website (http://bnci-horizon-2020.eu/database/data-sets). Once you have the files, you can use the following code snippet to load a specific participant's data:
import mne
# Set the path to the dataset directory
data_path = '<path_to_dataset_directory>'
# Load the raw EEG data for a specific participant
raw = mne.io.read_raw_gdf(data_path + '/A01T.gdf', preload=True)
Replace <path_to_dataset_directory> with the actual path to the directory where you've stored the dataset files. This code loads the EEG data for participant "A01" during the training session ("T").
2. Data Preprocessing: Refining the EEG Signals for P300 Detection
Raw EEG data is often a mixture of brain signals, artifacts, and noise. Before we can effectively detect the P300 component, we need to clean up the data and isolate the relevant frequencies.
Channel Selection: Focusing on the P300's Neighborhood
The P300 component is typically most prominent over the central-parietal region of the scalp. Therefore, we'll select channels that capture activity from this area. Commonly used channels for P300 detection include:
- Cz: The electrode located at the vertex of the head, directly over the central sulcus.
- Pz: The electrode located over the parietal lobe, slightly posterior to Cz.
- Surrounding Electrodes: Additional electrodes surrounding Cz and Pz, such as CPz, FCz, and P3/P4, can also provide valuable information.
These electrodes are chosen because they tend to be most sensitive to the positive voltage deflection that characterizes the P300 response.
# Select the desired channels
channels = ['Cz', 'Pz', 'CPz', 'FCz', 'P3', 'P4']
# Create a new raw object with only the selected channels
raw_selected = raw.pick_channels(channels)
Filtering: Tuning into the P300 Frequency
The P300 component is a relatively slow brainwave, typically occurring in the frequency range of 0.1 Hz to 10 Hz. Filtering helps us remove unwanted frequencies outside this range, enhancing the signal-to-noise ratio for P300 detection.
We'll apply a band-pass filter to the selected EEG channels, using cutoff frequencies of 0.1 Hz and 10 Hz:
# Apply a band-pass filter from 0.1 Hz to 10 Hz
raw_filtered = raw_selected.filter(l_freq=0.1, h_freq=10)
This filter removes slow drifts (below 0.1 Hz) and high-frequency noise (above 10 Hz), allowing the P300 component to stand out more clearly.
Artifact Removal (Optional): Combating Unwanted Signals
Depending on the quality of the EEG data and the presence of artifacts, we might need to apply additional artifact removal techniques. Independent Component Analysis (ICA) is a powerful method for separating independent sources of activity in EEG recordings. If the BNCI Horizon 2020 dataset contains significant artifacts, we can use ICA to identify and remove components related to eye blinks, muscle activity, or other sources of interference.
3. Epoching and Averaging: Isolating the P300 Response
To capture the brain's response to specific stimuli, we'll create epochs, time-locked segments of EEG data centered around events of interest.
Defining Epochs: Capturing the P300 Time Window
We'll define epochs around both target stimuli (the letters the user is focusing on) and non-target stimuli (all other letters). The epoch time window should capture the P300 response, typically occurring between 300 and 500 milliseconds after the stimulus onset. We'll use a window of -200 ms to 800 ms to include a baseline period and capture the full P300 waveform.
# Define event IDs for target and non-target stimuli (refer to dataset documentation)
event_id = {'target': 1, 'non-target': 0}
# Set the epoch time window
tmin = -0.2 # 200 ms before stimulus onset
tmax = 0.8 # 800 ms after stimulus onset
# Create epochs
epochs = mne.Epochs(raw_filtered, events, event_id, tmin, tmax, baseline=(-0.2, 0), preload=True)
Baseline Correction: Removing Pre-Stimulus Bias
Baseline correction involves subtracting the average activity during the baseline period (-200 ms to 0 ms) from each epoch. This removes any pre-existing bias in the EEG signal, ensuring that the measured response is truly due to the stimulus.
Averaging Evoked Responses: Enhancing the P300 Signal
To enhance the P300 signal and reduce random noise, we'll average the epochs for target and non-target stimuli separately. This averaging process reveals the event-related potential (ERP), a characteristic waveform reflecting the brain's response to the stimulus.
# Average the epochs for target and non-target stimuli
evoked_target = epochs['target'].average()
evoked_non_target = epochs['non-target'].average()
4. Feature Extraction: Quantifying the P300
Selecting Features: Capturing the P300's Signature
The P300 component is characterized by a positive voltage deflection peaking around 300-500 ms after the stimulus onset. We'll select features that capture this signature:
- Peak Amplitude: The maximum amplitude of the P300 component.
- Mean Amplitude: The average amplitude within a specific time window around the P300 peak.
- Latency: The time it takes for the P300 component to reach its peak amplitude.
These features provide a quantitative representation of the P300 response, allowing us to train a classifier to distinguish between target and non-target stimuli.
Extracting Features: From Waveforms to Numbers
We can extract these features from the averaged evoked responses using MNE-Python's functions:
# Extract peak amplitude
peak_amplitude_target = evoked_target.get_data().max(axis=1)
peak_amplitude_non_target = evoked_non_target.get_data().max(axis=1)
# Extract mean amplitude within a time window (e.g., 300 ms to 500 ms)
mean_amplitude_target = evoked_target.crop(tmin=0.3, tmax=0.5).get_data().mean(axis=1)
mean_amplitude_non_target = evoked_non_target.crop(tmin=0.3, tmax=0.5).get_data().mean(axis=1)
# Extract latency of the P300 peak
latency_target = evoked_target.get_peak(tmin=0.3, tmax=0.5)[1]
latency_non_target = evoked_non_target.get_peak(tmin=0.3, tmax=0.5)[1]
5. Classification: Training the Brainwave Decoder
Choosing a Classifier: LDA for P300 Speller Decoding
Linear Discriminant Analysis (LDA) is a suitable classifier for P300 spellers due to its simplicity, efficiency, and ability to handle high-dimensional data. It seeks to find a linear combination of features that best separates the classes (target vs. non-target).
Training the Model: Learning from Brainwaves
We'll train the LDA classifier using the extracted features:
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis
# Create an LDA object
lda = LinearDiscriminantAnalysis()
# Combine the features into a data matrix
X = np.vstack((peak_amplitude_target, peak_amplitude_non_target,
mean_amplitude_target, mean_amplitude_non_target,
latency_target, latency_non_target)).T
# Create a label vector (1 for target, 0 for non-target)
y = np.concatenate((np.ones(len(peak_amplitude_target)), np.zeros(len(peak_amplitude_non_target))))
# Train the LDA model
lda.fit(X, y)
Feature selection plays a crucial role here. By choosing features that effectively capture the P300 response, we improve the classifier's ability to distinguish between target and non-target stimuli.
6. Visualization: Validating Our Progress
Visualizing Preprocessed Data and P300 Responses
Visualizations help us understand the data and validate our preprocessing steps:
- Plot Averaged Epochs: Use evoked_target.plot() and evoked_non_target.plot() to visualize the average target and non-target epochs, confirming the presence of the P300 component in the target epochs.
- Topographical Plot: Use evoked_target.plot_topomap() to visualize the scalp distribution of the P300 component, ensuring it's most prominent over the expected central-parietal region.
Performance Evaluation: Assessing Speller Accuracy
Now that we've built our P300 speller, it's crucial to evaluate its performance. We need to assess how accurately it can distinguish between target and non-target stimuli, and consider practical factors that might influence its usability in real-world settings.
Cross-Validation: Ensuring Robustness and Generalizability
To obtain a reliable estimate of our speller's performance, we'll use k-fold cross-validation. This technique involves splitting the data into k folds, training the model on k-1 folds, and testing it on the remaining fold. Repeating this process k times, with each fold serving as the test set once, gives us a robust measure of the model's ability to generalize to unseen data.
from sklearn.model_selection import cross_val_score
# Perform 5-fold cross-validation
scores = cross_val_score(lda, X, y, cv=5)
# Print the average accuracy across the folds
print("Average accuracy: %0.2f" % scores.mean())
This code performs 5-fold cross-validation using our trained LDA classifier and prints the average accuracy across the folds.
Metrics for P300 Spellers: Beyond Accuracy
While accuracy is a key metric for P300 spellers, indicating the proportion of correctly classified stimuli, other metrics provide additional insights:
- Information Transfer Rate (ITR): Measures the speed of communication, taking into account the number of possible choices and the accuracy of selection. A higher ITR indicates a faster and more efficient speller.
Practical Considerations: Bridging the Gap to Real-World Use
Several practical factors can influence the performance and usability of P300 spellers:
- User Variability: P300 responses can vary significantly between individuals due to factors like age, attention, and neurological conditions. To address this, personalized calibration is crucial, where the speller is adjusted to each user's unique brain responses. Adaptive algorithms can also be employed to continuously adjust the speller based on the user's performance.
- Fatigue and Attention: Prolonged use can lead to fatigue and decreased attention, affecting P300 responses and speller accuracy. Strategies to mitigate this include incorporating breaks, using engaging stimuli, and employing algorithms that can detect and adapt to changes in user state.
- Training Duration: The amount of training a user receives can impact their proficiency with the speller. Sufficient training is essential for users to learn to control their P300 responses and achieve optimal performance.
Empowering Communication with P300 Spellers
We've successfully built a P300 speller, witnessing firsthand the power of EEG, signal processing, and machine learning to create a functional BCI application. These spellers hold immense potential as a communication tool, enabling individuals with severe motor impairments to express themselves, connect with others, and participate more fully in the world.
Further Reading and Resources
- Review article: Pan J et al. Advances in P300 brain-computer interface spellers: toward paradigm design and performance evaluation. Front Hum Neurosci. 2022 Dec 21;16:1077717. doi: 10.3389/fnhum.2022.1077717. PMID: 36618996; PMCID: PMC9810759.
- Dataset: BNCI Horizon 2020 P300 dataset: http://bnci-horizon-2020.eu/database/data-sets
- Tutorial: PyQt documentation for GUI development (optional): https://doc.qt.io/qtforpython/
Future Directions: Advancing P300 Speller Technology
The field of P300 speller development is constantly evolving. Emerging trends include:
- Deep Learning: Applying deep learning algorithms to improve P300 detection accuracy and robustness.
- Multimodal BCIs: Combining EEG with other brain imaging modalities (e.g., fNIRS) or physiological signals (e.g., eye tracking) to enhance speller performance.
- Hybrid Approaches: Integrating P300 spellers with other BCI paradigms (e.g., motor imagery) to create more flexible and versatile communication systems.
Next Stop: Motor Imagery BCIs
In the next blog post, we'll explore motor imagery BCIs, a fascinating paradigm where users control devices by simply imagining movements. We'll dive into the brain signals associated with motor imagery, learn how to extract features, and build a classifier to decode these intentions.